How Can I Run Java Program
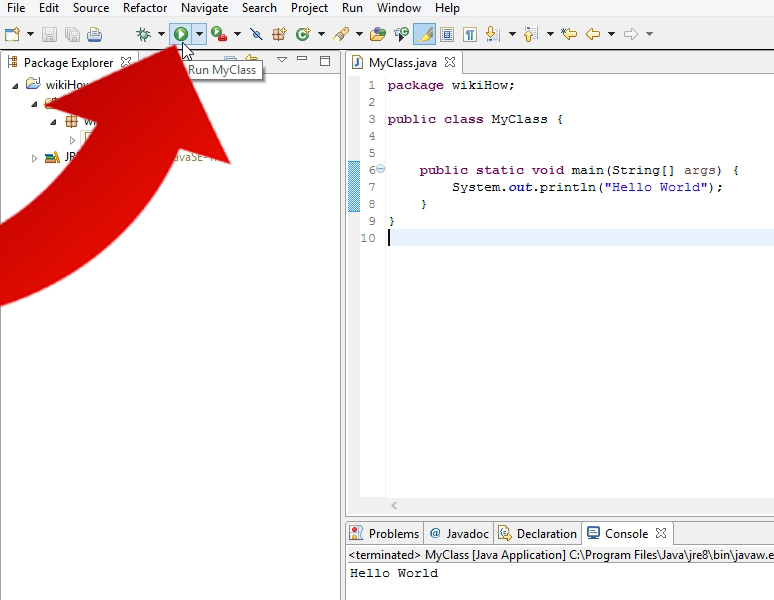
Java is one of the most popular and widely used programming languages, powering countless applications and systems worldwide. Whether you're a beginner taking your first steps into programming or an experienced developer, understanding how to run a Java program is essential. In this comprehensive guide, we will delve into the process of executing Java code, covering various methods and considerations to ensure a smooth and successful execution.
Getting Started with Java

Before we dive into running Java programs, let’s ensure you have the necessary prerequisites in place.
Java Development Kit (JDK)
The first step is to install the Java Development Kit (JDK), which provides the Java Runtime Environment (JRE) and the tools needed to compile and run Java applications. You can download the latest JDK version from the Oracle website or choose an open-source distribution like AdoptOpenJDK. Follow the installation instructions specific to your operating system.
Integrated Development Environment (IDE)
While not strictly necessary, using an Integrated Development Environment (IDE) can greatly enhance your Java development experience. Popular IDEs for Java include Eclipse, IntelliJ IDEA, and Visual Studio Code with the Java Extension Pack. These IDEs provide code editing, debugging, and compilation features, making your Java programming journey more efficient and enjoyable.
Running a Java Program

Now that you have the JDK and an IDE (if desired) installed, let’s explore the different methods to run a Java program.
Method 1: Using the Command Line
One of the simplest ways to run a Java program is through the command line. Follow these steps:
- Compile the Java Code: Open a terminal or command prompt and navigate to the directory where your Java source code file (e.g.,
MyProgram.java
) is located. Compile the code using thejavac
command:
javac MyProgram.java
This will generate a .class
file containing the compiled bytecode.
- Run the Java Program: Execute the compiled program using the
java
command:
java MyProgram
If your program requires arguments, you can pass them as follows:
java MyProgram arg1 arg2
Replace MyProgram
with the actual name of your Java class, and ensure the class contains a main
method with the desired arguments.
Method 2: Running from an IDE
If you’re using an IDE, the process of running a Java program becomes even more streamlined.
- Open the Java Project: Launch your IDE and open the Java project containing your source code.
- Set the Main Class: In the project settings or build configuration, specify the class containing the
main
method as the main class or entry point. - Run the Program: Use the IDE's run functionality, often accessible through a dedicated button or a keyboard shortcut. The IDE will compile and execute your program, providing a convenient debugging environment.
Method 3: Using a Build Tool (Maven or Gradle)
For more complex projects or when working with build automation, tools like Maven and Gradle can simplify the build and execution process.
- Configure the Build Tool: In your project's build configuration file (e.g.,
pom.xml
for Maven orbuild.gradle
for Gradle), define the dependencies and build settings. - Compile and Run: Execute the appropriate commands to compile and run your Java program. For Maven, use:
mvn clean package java -jar target/myapp-1.0.0.jar
For Gradle, you can use:
gradle clean build java -jar build/libs/myapp-1.0.0.jar
Replace myapp
with your project's actual name.
Troubleshooting and Common Issues
While running Java programs is generally straightforward, you might encounter a few common issues. Here are some troubleshooting tips:
Java Environment Setup
- Path Configuration: Ensure that the
java
andjavac
commands are accessible from your command line by adding the JDK’sbin
directory to your system’s PATH variable. - Java Version: Verify that you are using the correct Java version by checking the output of
java -version
andjavac -version
commands.
Compilation Errors
If you encounter compilation errors, carefully review the error messages provided by the compiler. Common issues include syntax errors, missing semicolons, or incorrect class/method names. Ensure your code adheres to Java’s syntax and structure.
Runtime Exceptions
During execution, your Java program might throw runtime exceptions. These can be due to incorrect input handling, null pointer exceptions, or issues with the program logic. Proper error handling and debugging techniques are crucial to identifying and resolving such issues.
Conclusion
Running a Java program is a fundamental skill for any Java developer. Whether you prefer the command line, an IDE, or build tools, the process is straightforward once you have the necessary tools and configurations in place. Remember to troubleshoot any issues that arise and utilize the extensive documentation and community support available for Java development.
FAQs

Can I run a Java program without compiling it first?
+No, Java programs need to be compiled into bytecode before they can be executed. The compilation step ensures that the Java code is converted into a format that can be understood by the Java Virtual Machine (JVM) at runtime.
What is the purpose of an IDE in Java development?
+An IDE, or Integrated Development Environment, provides a comprehensive set of tools and features to streamline Java development. It offers code editing, syntax highlighting, debugging capabilities, and project management, making the development process more efficient and productive.
How can I handle exceptions and errors in my Java program?
+Java provides robust exception handling mechanisms through the use of try-catch
blocks. By wrapping potential error-causing code within a try
block and catching the exceptions with a catch
block, you can gracefully handle errors and provide appropriate error messages or alternative execution paths.