How To Code For Linux
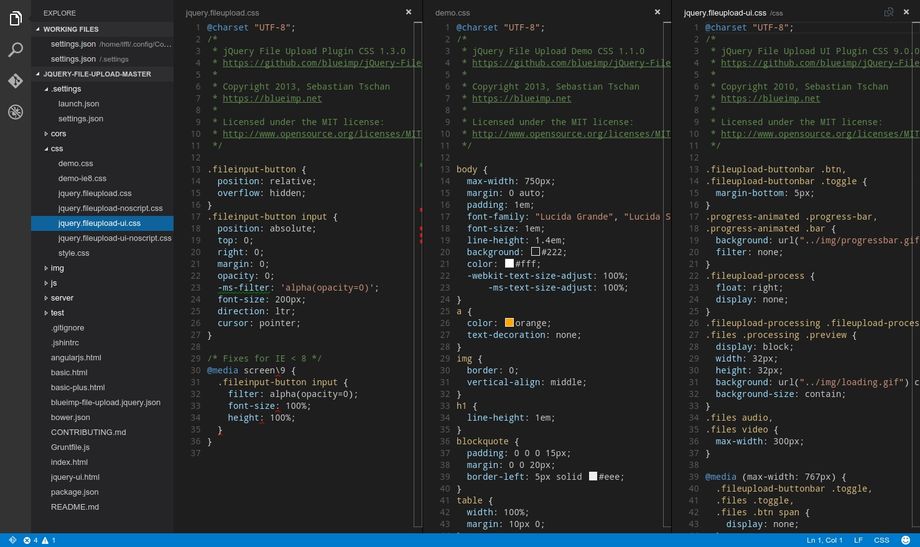
Welcome to the world of Linux, an operating system that has captivated millions of users and developers worldwide. In this comprehensive guide, we will explore the art of coding for Linux, a journey that will empower you to create innovative applications, explore open-source projects, and unleash your creativity within the Linux ecosystem. Whether you're a seasoned developer or a curious beginner, this article will provide you with the knowledge and tools to start coding for Linux and make the most of this powerful and versatile platform.
Understanding the Linux Ecosystem

Linux, a free and open-source operating system, has gained immense popularity among developers and tech enthusiasts. Its flexibility, stability, and vast community support make it an ideal platform for coding and software development. In this section, we’ll delve into the key aspects of the Linux ecosystem to set the foundation for your coding journey.
The Linux Philosophy
Linux was born out of the vision of a free and open-source operating system, where users have the freedom to modify and distribute the software. This philosophy has attracted a vibrant community of developers who contribute to its continuous improvement. The Linux ecosystem fosters collaboration, innovation, and a culture of sharing, making it an ideal environment for developers to thrive.
Linux Distributions
One of the unique characteristics of Linux is the plethora of distributions (or distros) available. Each distro offers a unique blend of software, package management systems, and user interfaces. Some popular distros include Ubuntu, Fedora, Debian, and Arch Linux. Choosing the right distro depends on your specific needs and preferences. For beginners, user-friendly distros like Ubuntu provide a smooth entry point, while more advanced users might opt for distros like Arch Linux for greater customization and control.
Package Management Systems
Linux distributions utilize package management systems to install, update, and remove software. These systems simplify the process of managing software dependencies and ensure a smooth and secure software environment. Some common package managers include APT (Advanced Packaging Tool) for Debian-based distros, and Yum (Yellowdog Updater, Modified) for Red Hat-based distros. Understanding package management is crucial for efficient software development and deployment on Linux.
The Linux Command Line
The Linux command line, or terminal, is a powerful tool for developers. It provides a direct interface to the operating system, allowing you to perform a wide range of tasks, from navigating the file system to executing complex scripts. Mastering the command line is essential for efficient coding and system administration tasks. Linux offers a rich set of command-line utilities and tools that can streamline your workflow.
Setting Up Your Development Environment

To begin coding for Linux, you’ll need to set up a suitable development environment. This section will guide you through the process, ensuring you have the necessary tools and configurations in place to start your coding journey.
Choosing Your IDE
An Integrated Development Environment (IDE) is a powerful tool that provides a comprehensive set of features for coding and debugging. For Linux, there are numerous IDEs available, each with its own strengths and weaknesses. Some popular choices include:
- Visual Studio Code: A lightweight and versatile IDE, offering a wide range of extensions and features. It is highly customizable and supports multiple programming languages.
- Eclipse: A mature and feature-rich IDE, known for its powerful plugins and support for various programming languages. Eclipse is particularly popular for Java development.
- Atom: A hackable text editor built with web technologies. Atom offers a smooth development experience and is highly customizable through its package system.
Installing Essential Tools
In addition to an IDE, you’ll need to install a range of tools and libraries to support your coding projects. This may include compilers, interpreters, and libraries specific to the programming languages you intend to work with. Some essential tools to consider are:
- GCC (GNU Compiler Collection): A suite of compilers for various programming languages, including C, C++, and Fortran. GCC is widely used and supported across Linux distributions.
- Python: A versatile and popular programming language, offering a wide range of libraries and frameworks for various domains, from web development to data science.
- Node.js: A powerful runtime environment for JavaScript, enabling you to build server-side applications, command-line tools, and more. Node.js is widely used in modern web development.
Configuring Your Environment
Once you have your IDE and tools installed, it’s time to configure your development environment to suit your needs. This may involve setting up code formatting preferences, keyboard shortcuts, and other customizations to enhance your productivity. Additionally, you’ll want to ensure your environment is properly configured for the specific programming languages and frameworks you plan to work with.
Coding with Linux: A Practical Guide
Now that your development environment is set up, it’s time to dive into the world of coding for Linux. In this section, we’ll provide a step-by-step guide to help you get started with your first Linux-based project.
Choosing a Programming Language
Linux supports a vast array of programming languages, each with its own strengths and use cases. Some popular choices include:
- C and C++: Low-level languages known for their performance and control, making them ideal for system-level programming and performance-critical applications.
- Python: A high-level, versatile language, perfect for rapid prototyping, data analysis, and automation tasks.
- JavaScript: The language of the web, enabling you to build dynamic web applications, interactive user interfaces, and server-side applications.
- Go (Golang): A modern, efficient language developed by Google, designed for building scalable network services and distributed systems.
Creating Your First Project
Let’s walk through the process of creating a simple project in your chosen programming language. For this example, we’ll use Python to build a basic web scraper.
- Install Python: If you haven’t already, install Python on your Linux system. You can do this through your package manager or by downloading the Python installer from the official website.
- Set Up a Virtual Environment: It’s good practice to create a virtual environment for your project. This isolates your project’s dependencies and ensures a clean development environment. You can use tools like venv or virtualenv to create virtual environments.
- Install Required Libraries: For web scraping, you’ll need a library like BeautifulSoup and Requests. You can install these libraries using pip, Python’s package manager.
- Write Your Code: Create a new Python file and write your web scraping code. Here’s a basic example:
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
# Extract and print data
for link in soup.find_all("a"):
print(link.get("href"))
- Run Your Code: Save your Python file and run it using the python command. You should see the extracted links from the web page printed to the console.
Exploring Open-Source Projects
Linux is deeply rooted in the open-source community, and there are countless open-source projects to explore and contribute to. Contributing to open-source projects not only enhances your coding skills but also allows you to give back to the community. Here are some steps to get started with open-source contributions:
- Find a Project: Browse through platforms like GitHub, GitLab, or Bitbucket to find open-source projects that align with your interests and skill level. Look for projects with clear contribution guidelines and an active community.
- Read the Documentation: Familiarize yourself with the project’s documentation, including its code structure, development guidelines, and contribution process.
- Choose a Task: Look for issues or tasks labeled as “good first issues” or “beginner-friendly.” These are often smaller, more manageable tasks suitable for newcomers.
- Fork and Clone: Fork the project’s repository to your own GitHub account and then clone it to your local machine.
- Make Changes: Work on the chosen task, making the necessary code changes and improvements. Ensure your code follows the project’s coding standards and best practices.
- Create a Pull Request: Once your changes are ready, create a pull request (PR) to propose your changes to the project’s maintainers. Provide a clear and detailed description of your changes and why they are beneficial.
- Collaborate and Iterate: Engage with the project’s maintainers and community members. Be open to feedback and suggestions, and iterate on your changes based on their input.
Advanced Linux Coding Techniques
As you advance in your Linux coding journey, you’ll encounter more complex scenarios and challenges. This section will explore some advanced techniques and best practices to take your Linux coding skills to the next level.
Containerization with Docker
Docker is a powerful containerization platform that allows you to package your applications and their dependencies into lightweight, portable containers. This ensures consistency across different environments and simplifies deployment. Here’s how to get started with Docker:
- Install Docker: Download and install Docker on your Linux system. You can find installation instructions on the official Docker website.
- Create a Dockerfile: A Dockerfile is a text file that contains instructions for building a Docker image. It specifies the base image, the necessary packages, and the command to run your application.
- Build and Run a Docker Image: Use the docker build command to build your Docker image, and then use docker run to launch your application in a container.
Continuous Integration and Deployment (CI/CD)
CI/CD pipelines automate the build, testing, and deployment process of your applications. This ensures that your code is always in a deployable state and facilitates efficient collaboration and deployment. Here’s a basic CI/CD pipeline setup using Jenkins, a popular CI/CD tool:
- Install Jenkins: Download and install Jenkins on your Linux system. You can follow the official Jenkins documentation for detailed instructions.
- Configure a CI/CD Pipeline: Create a Jenkins pipeline job and define the build, test, and deployment steps. This may involve setting up build triggers, configuring build environments, and defining deployment targets.
- Integrate with Version Control: Connect your Jenkins pipeline to your version control system (e.g., Git) to automatically trigger builds upon code changes.
- Test and Deploy: Jenkins will automatically build and test your application, and upon successful completion, deploy it to the specified target environment.
Security Best Practices
When coding for Linux, it’s crucial to follow security best practices to protect your applications and data. Here are some key practices to consider:
- Use Secure Communication Protocols: Ensure your applications use secure communication protocols like HTTPS for web applications and SSH for remote access.
- Implement Authentication and Authorization: Implement robust authentication mechanisms to ensure only authorized users can access your applications. Use tools like OAuth or JWT for token-based authentication.
- Encrypt Sensitive Data: Encrypt any sensitive data, such as user credentials or personal information, to protect it from unauthorized access.
- Regularly Update and Patch: Keep your Linux system and software up to date with the latest security patches and updates. This helps mitigate known vulnerabilities and security risks.
Conclusion

Coding for Linux opens up a world of possibilities, from building innovative applications to contributing to open-source projects. With its flexibility, stability, and vibrant community, Linux provides an ideal platform for developers to thrive. By following the steps outlined in this guide, you’ll be well on your way to becoming a proficient Linux coder. Remember, the Linux community is always ready to welcome and support new developers, so don’t hesitate to reach out and ask for help when needed.
What are some popular package managers for Linux?
+Some popular package managers for Linux include APT (Advanced Packaging Tool) for Debian-based distros, Yum (Yellowdog Updater, Modified) for Red Hat-based distros, and Pacman for Arch Linux. These package managers simplify the process of installing, updating, and removing software packages on Linux systems.
How can I contribute to open-source projects on Linux?
+Contributing to open-source projects on Linux involves finding a project that aligns with your interests and skill level, reading the project’s documentation and contribution guidelines, choosing a task or issue to work on, forking the project’s repository, making the necessary code changes, and creating a pull request to propose your changes to the project’s maintainers. Engaging with the project’s community and iterating on your changes based on feedback is also crucial.
What are some best practices for securing my Linux applications?
+To secure your Linux applications, it’s important to use secure communication protocols like HTTPS for web applications and SSH for remote access, implement robust authentication and authorization mechanisms, encrypt sensitive data, and regularly update and patch your Linux system and software to mitigate known vulnerabilities and security risks. Following these best practices will help protect your applications and data from unauthorized access and potential security threats.