Mastering Matlab: 5 If-Else Tips

MATLAB, a versatile and powerful programming language, offers a wide range of capabilities for data analysis, visualization, and algorithm development. One of its fundamental features is the ability to execute conditional statements, allowing programmers to control the flow of their code based on certain conditions. Among these, the if-else statement is a cornerstone of MATLAB programming, enabling users to create dynamic and adaptable code. In this comprehensive guide, we delve into the world of MATLAB's if-else statements, offering expert insights and practical tips to help you master this essential tool.
Understanding MATLAB’s If-Else Statements
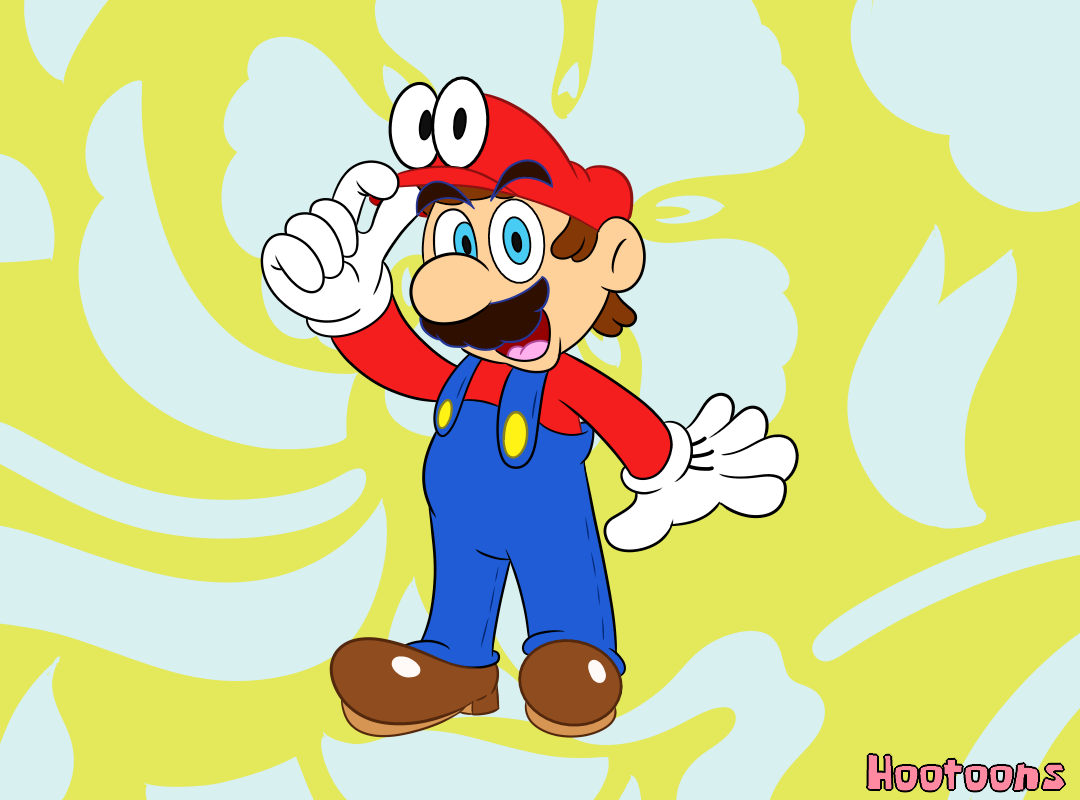
The if-else statement in MATLAB is a conditional construct that allows you to execute specific blocks of code based on whether a condition is true or false. This fundamental control flow mechanism is essential for building dynamic and interactive MATLAB programs. By evaluating a condition, MATLAB determines whether to execute the code within the if block, the else block, or both, depending on the outcome of the condition.
Here's a basic example of an if-else statement in MATLAB:
x = 10;
if x > 5
disp('x is greater than 5');
else
disp('x is less than or equal to 5');
end
In this example, the if block is executed because the condition x > 5
is true, resulting in the output 'x is greater than 5'. If x
were less than or equal to 5, the else block would be executed instead.
5 Expert Tips for Mastering MATLAB’s If-Else Statements

MATLAB’s if-else statements are a powerful tool, but like any programming construct, they can be used more effectively with the right techniques. Here are five expert tips to help you master MATLAB’s if-else statements and elevate your programming skills:
1. Utilize Nested If-Else Statements
MATLAB allows for nested if-else statements, which can be incredibly useful when you need to make multiple decisions based on different conditions. By nesting if-else statements, you can create a hierarchical decision-making structure within your code. This is particularly beneficial when dealing with complex logic or when you want to perform different actions based on various outcomes.
Consider the following example, which demonstrates nested if-else statements:
temperature = 25;
if temperature > 30
disp('It''s very hot outside!');
else
if temperature > 20
disp('It''s pleasantly warm.');
else
disp('It''s quite cold.');
end
end
In this example, the outer if statement checks if the temperature
is greater than 30. If it is, the corresponding message is displayed. If not, the code moves to the else block, where another if statement checks if the temperature is greater than 20. Depending on the outcome, the appropriate message is displayed.
2. Employ Elseif for Multiple Conditions
The elseif statement in MATLAB is a powerful tool when you have multiple conditions to check. It allows you to evaluate a series of conditions and execute the corresponding code block based on the first condition that evaluates to true. This can simplify your code and make it more readable, especially when dealing with complex logic.
Here's an example that demonstrates the use of elseif:
grade = 85;
if grade >= 90
disp('A - Excellent!');
elseif grade >= 80
disp('B - Good job!');
elseif grade >= 70
disp('C - Room for improvement.');
else
disp('D - Needs attention.');
end
In this example, the code checks the grade
variable against a series of conditions using elseif statements. The first condition that evaluates to true triggers the corresponding code block, and the program execution continues from there.
3. Optimize with Switch-Case Statements
In some cases, using a switch-case statement can be more efficient and readable than a series of if-else or elseif statements, especially when you have a large number of discrete conditions to evaluate. The switch-case statement in MATLAB allows you to specify a variable and then define a set of cases based on different values of that variable.
Here's an example of a switch-case statement:
shape = 'circle';
switch shape
case 'circle'
disp('It''s a circle.');
case 'square'
disp('It''s a square.');
case 'triangle'
disp('It''s a triangle.');
otherwise
disp('Unknown shape.');
end
In this example, the switch statement evaluates the shape
variable, and the corresponding case block is executed based on the value of the shape
. The otherwise block is a catch-all for any values not explicitly listed in the case statements.
4. Combine with Logical Operators
MATLAB provides a range of logical operators that you can use to create more complex conditions within your if-else statements. These operators allow you to combine multiple conditions using logical AND (&&
), logical OR (||
), and logical NOT (!
) operations.
For example, you can use the logical AND operator to check if two conditions are both true before executing a specific block of code:
x = 10;
y = 20;
if x > 5 && y > 15
disp('Both conditions are true.');
else
disp('At least one condition is false.');
end
In this example, the code checks if both x
is greater than 5 and y
is greater than 15. If both conditions are true, the corresponding message is displayed.
5. Leverage Vectorized Operations
MATLAB is renowned for its support of vectorized operations, which can significantly improve the performance and readability of your code. Instead of using loops to process each element of an array or matrix, you can often perform vectorized operations that operate on the entire array at once. This can be especially useful when combined with if-else statements.
For instance, consider a scenario where you want to check if any element in a vector is greater than a certain value. Instead of using a loop, you can use MATLAB's vectorized operations to achieve this more efficiently:
values = [10 20 15 5 30];
threshold = 15;
if any(values > threshold)
disp('At least one value is greater than the threshold.');
else
disp('All values are less than or equal to the threshold.');
end
In this example, the any
function checks if any element in the values
vector is greater than the threshold
, returning true if so. This approach is more concise and efficient than using a loop to iterate through each element.
Conclusion
MATLAB’s if-else statements are a fundamental tool for building dynamic and interactive programs. By understanding and utilizing the tips outlined above, you can elevate your MATLAB programming skills and write more efficient, readable, and powerful code. Whether it’s through nested if-else statements, elseif constructs, switch-case statements, logical operators, or vectorized operations, MATLAB provides a wealth of tools to help you master conditional programming.
What are some common mistakes to avoid when using if-else statements in MATLAB?
+Common mistakes to avoid include forgetting the end
keyword to terminate the if-else block, using incorrect indentation or spacing, and not properly nesting if-else statements. Additionally, be cautious of infinite loops, which can occur if the conditions within an if-else statement are not properly structured.
Can I use multiple else blocks in an if-else statement in MATLAB?
+No, MATLAB only allows one else block per if statement. If you need to handle multiple conditions, consider using elseif statements or a switch-case statement instead.
Are there any performance considerations when using if-else statements in MATLAB?
+Yes, while if-else statements are essential for control flow, they can impact performance if used excessively or in a way that hinders vectorization. Whenever possible, leverage MATLAB’s vectorized operations to improve performance and code readability.